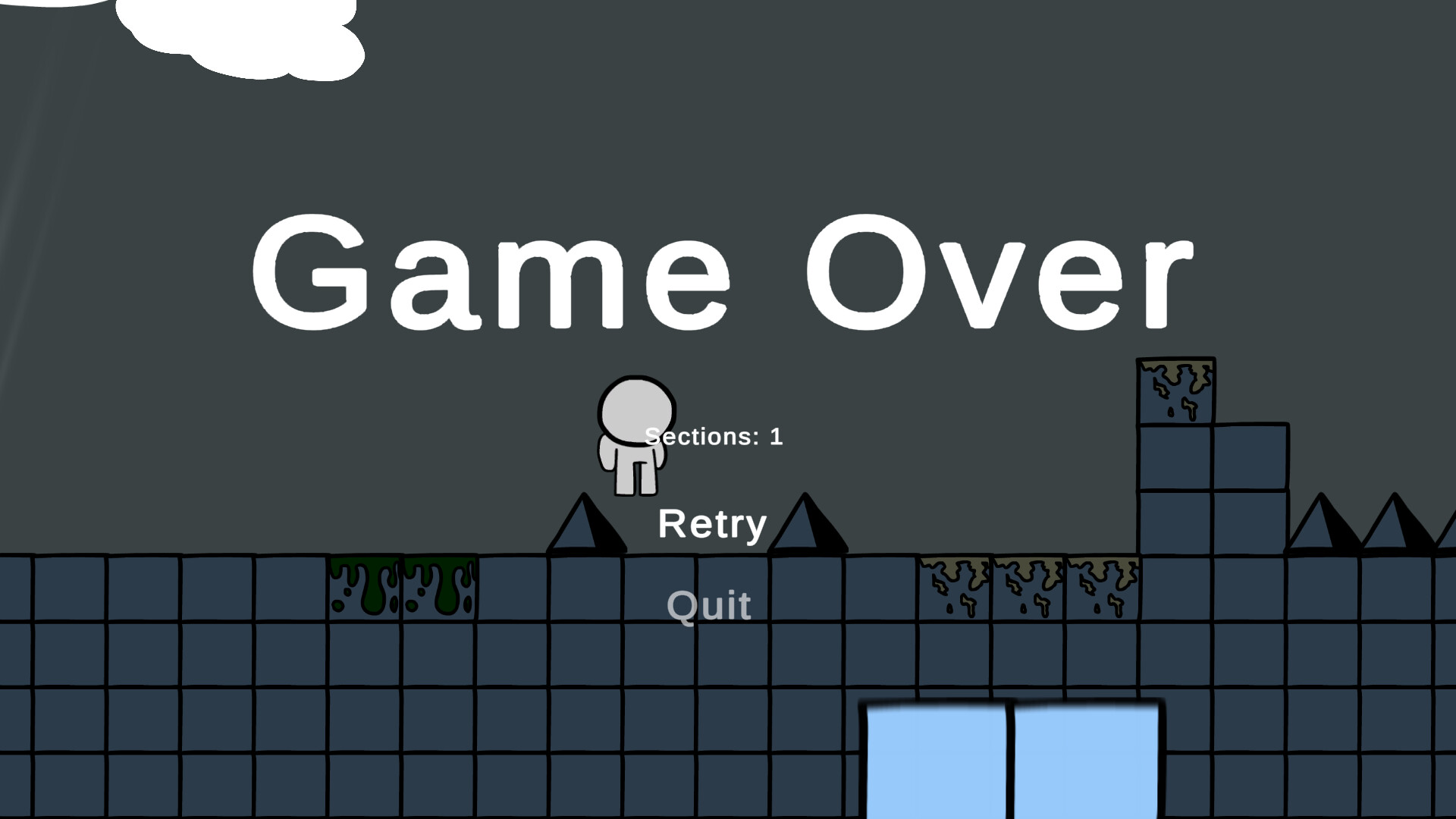
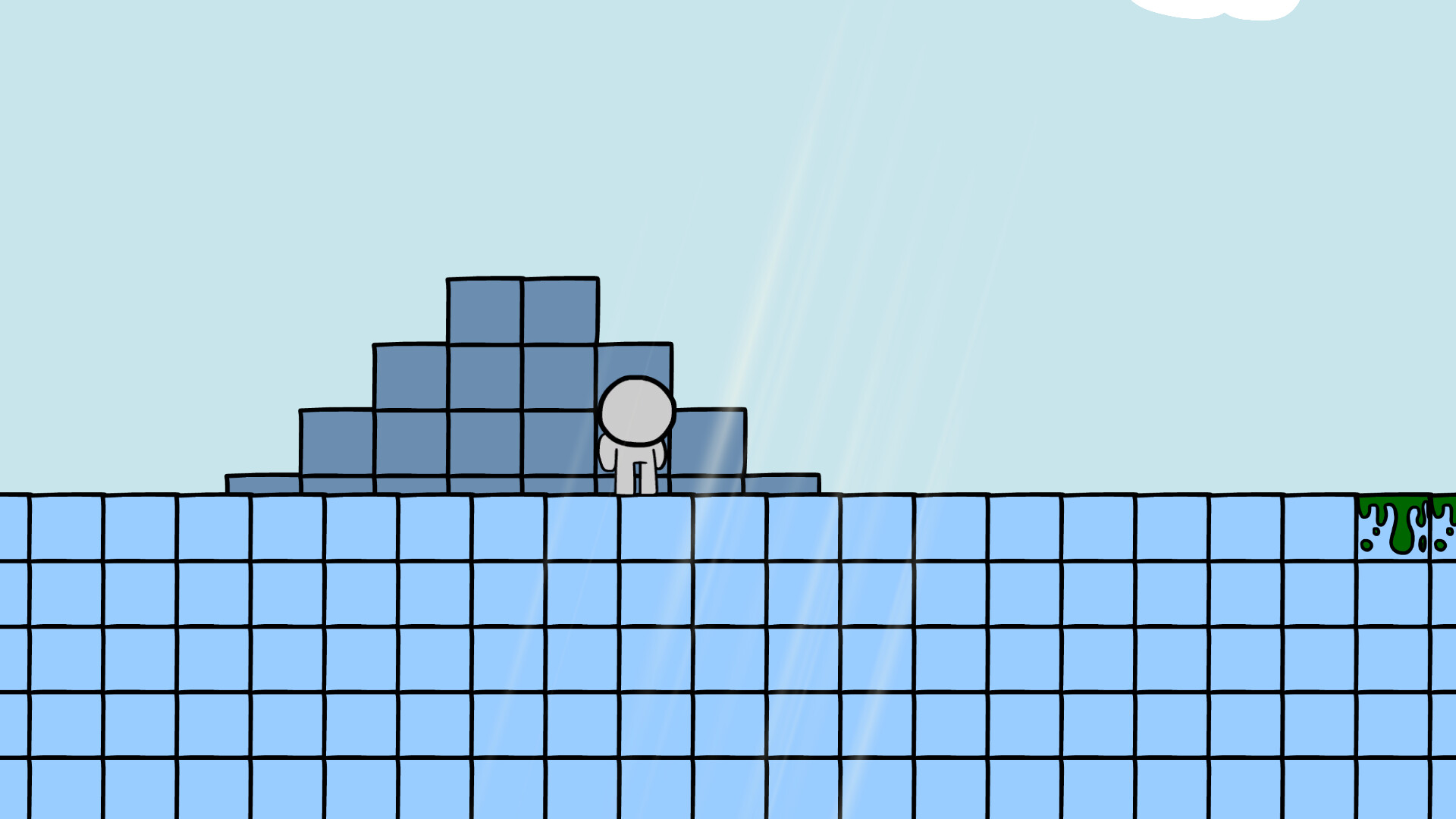
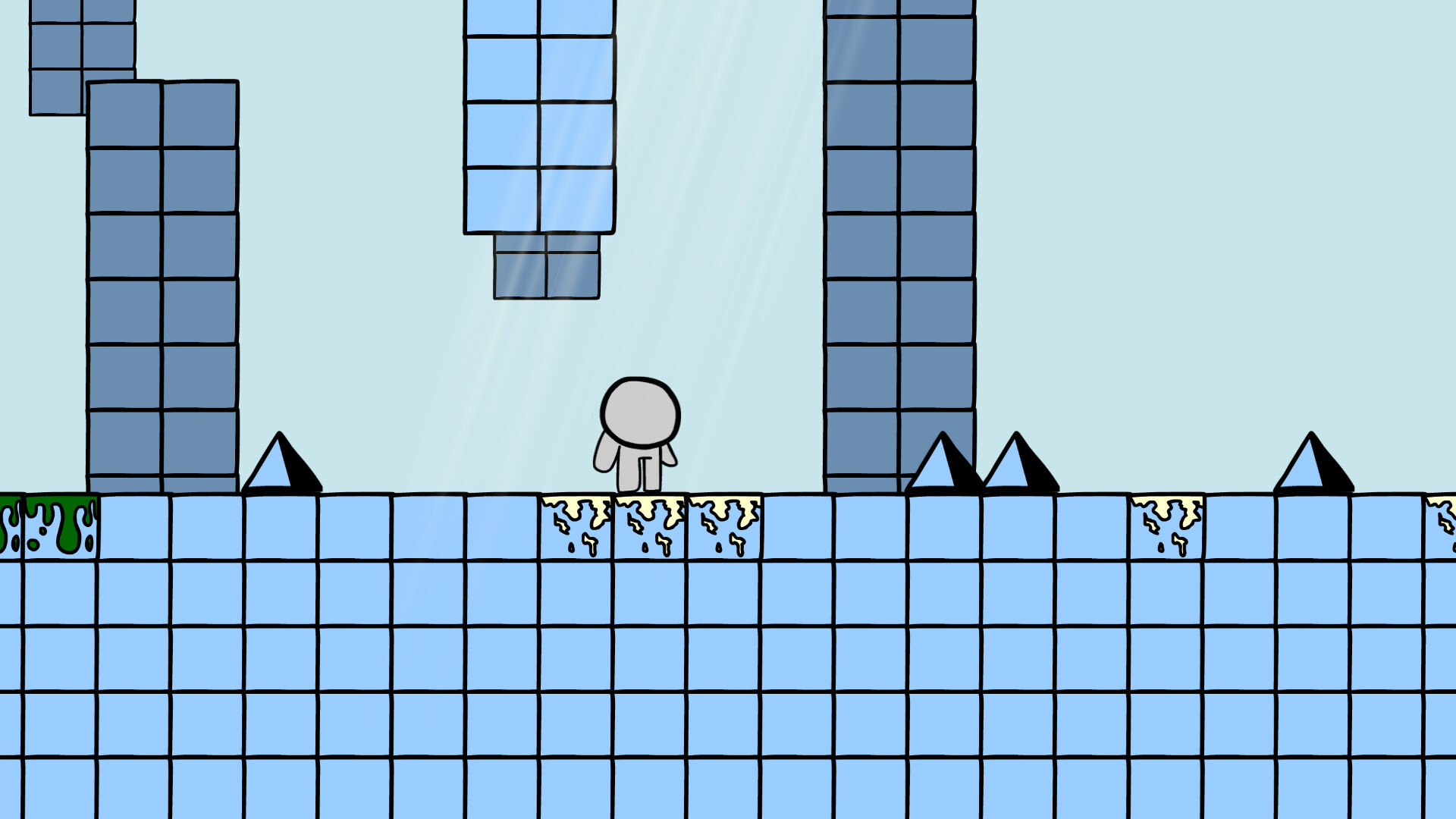
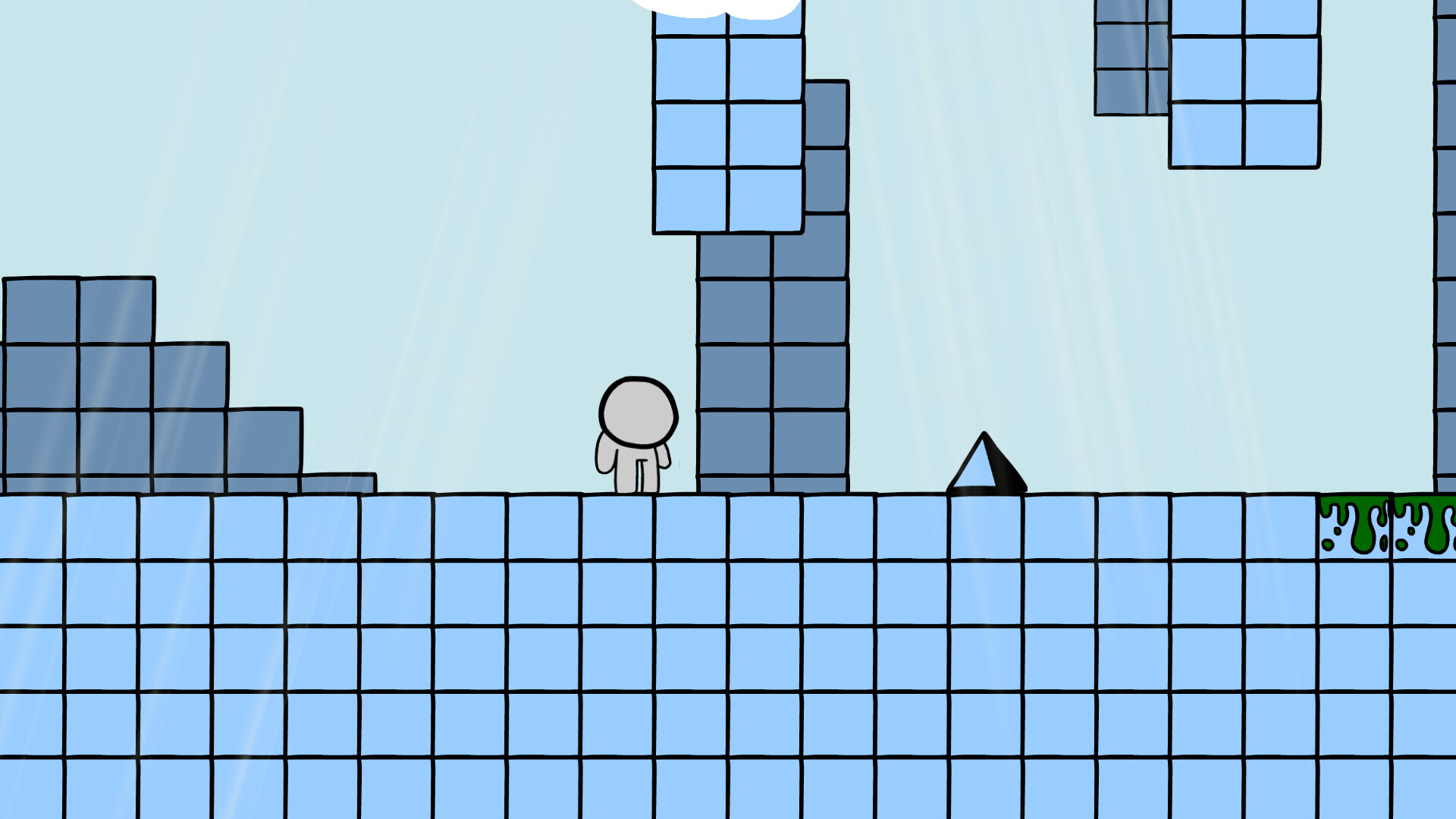
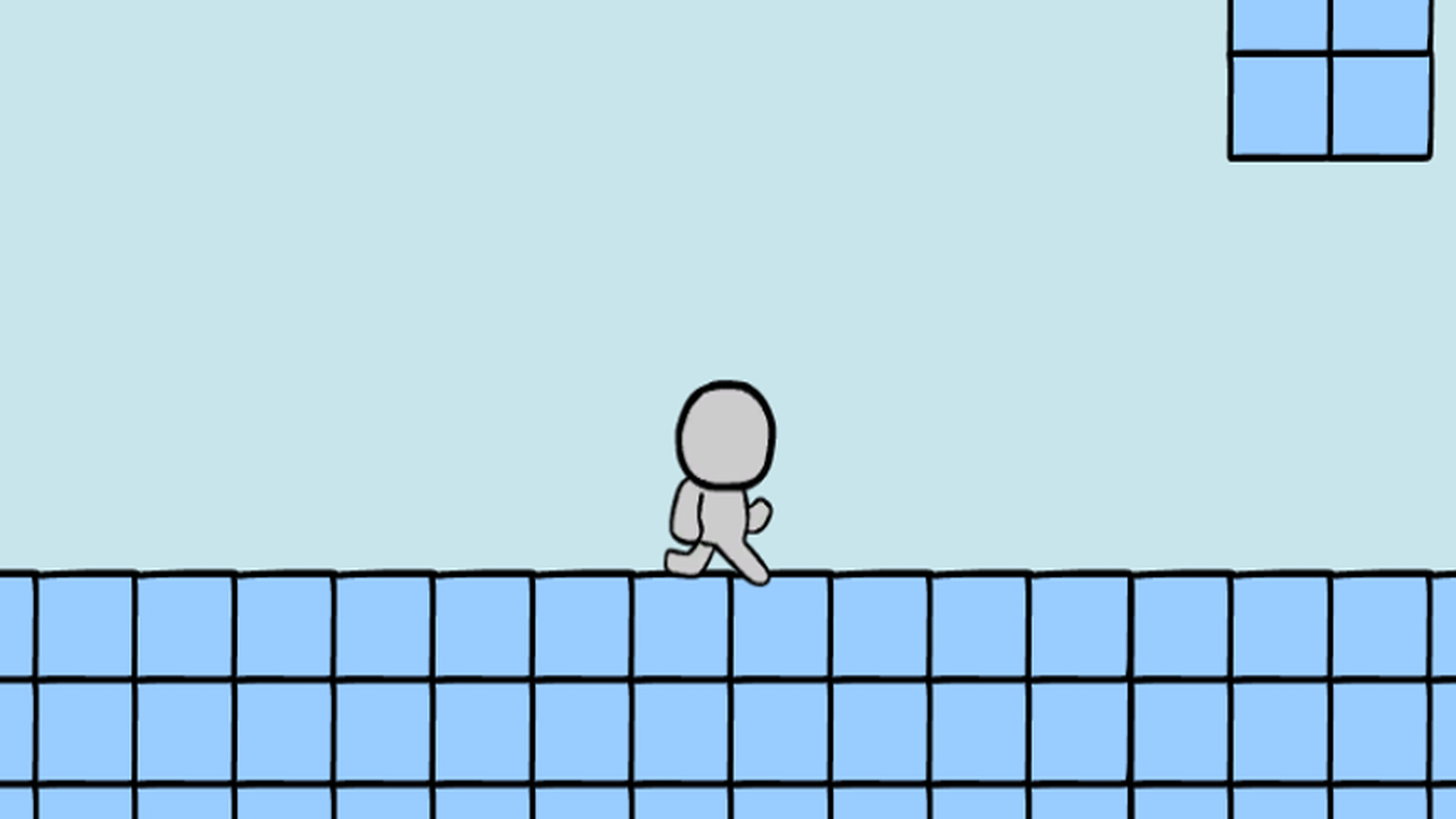
🌟 Special thanks to our amazing supporters:
✨ $10 Tier: [Geeks Love Detail]
🌈 $5 Tier: [Arch Toasty][Benedikt][David Martínez Martí]
- Fixed multiple bugs, including sections not syncing in multiplayer mode - You can now host games multiple times without restarting Speedrush Please Update at your convenience.
Hello everyone! Here are the improvements in this update for mod developers: - Collision capabilities - More advanced getters and setters - More callbacks - Bug fixes - Audio capabilities - Enumerations - And more! The API is being updated as of right now, check back in a few hours! Please Update at your convenience.
- Fixed Settings menu on desktop platforms Please Update at your convenience.
- Fixed sprites not destroying properly (Modding) - Fixed the singleplayer gameover screen not working on mobile devices - Performance fixes - Update to latest Unity version Please Update at your convenience.
- Android and FireOS Support (Both available on their respective app stores) - Update to latest Unity version Please Update at your convenience.
- Fixed a font issue in the mode selection screen - Fixed a control panel bug where mod icons would not be added Please Update at your convenience.
- Tweaked Ui on the title screen - Added a version counter on the title screen Please Update at your convenience.
- Minor cosmetic UI tweaks - Nametags on players Please Update at your convenience.
- Added helpful function parameters for Lua callbacks Please Update at your convenience.
- Fixed achievements only being awarded after leaving the game - Fixed the game freezing if you get eliminated while dashing This should be the last Bug fix update for awhile Please Update at your convenience.
- Fixed time stopping when exiting the Game Over screen - Changed 'setSpriteWidth' to 'setSpriteSize' for Lua scripts Please Update at your convenience.
- Fixed Lua's 'print' function not writing to the log file. Please Update at your convenience.
- Fixed a bug where sprites would only show on the host's perspective - You can now print a message to all clients using Lua scripts Please Update at your convenience.
- Fixed sprite width not applying for Lua scripts Please Update at your convenience.
I've fixed multiple bugs, one of which being that instead of the host being able to load mods, the player who last joined the server had access to the control panel. I've also fixed some alignment issues on some UI elements, such as the game over and mode selection menu screens. Please Update at your convenience.
In this update, officially supported modding and a few other changes have been added to Speedrush! However, unlike many other games which support modding using the C# programming language, Speedrush uses the Lua programming language, which is much easier to learn, even if you've never coded before. As of now, the API is in construction, however it should be done in a day or two. I've also changed the game's font to a more fitting one and added a "Modder" achievement, which you will be awarded if and when you decide to load a mod into your game. Keep in mind: - Speedrush mods can be loaded using the Control Panel, which is accessed by pressing the "Up" arrow key only on a server. - A single speedrush mod will affect all the players the the said server. - Only the host of the server can load mods. With that said: Enjoy Version 1.1 - The Modding Update!
[ 5945 ]
[ 1934 ]
[ 1837 ]