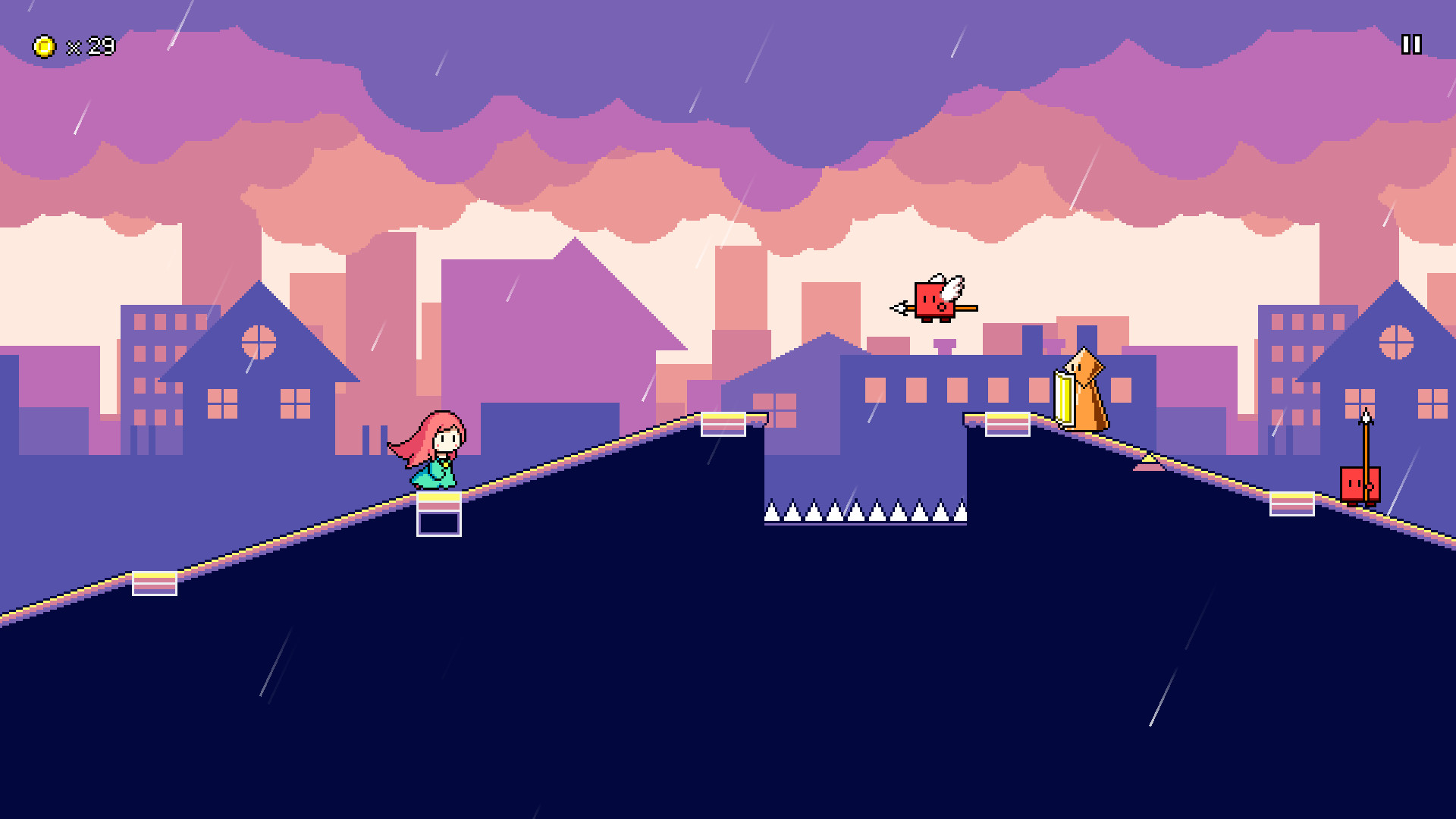
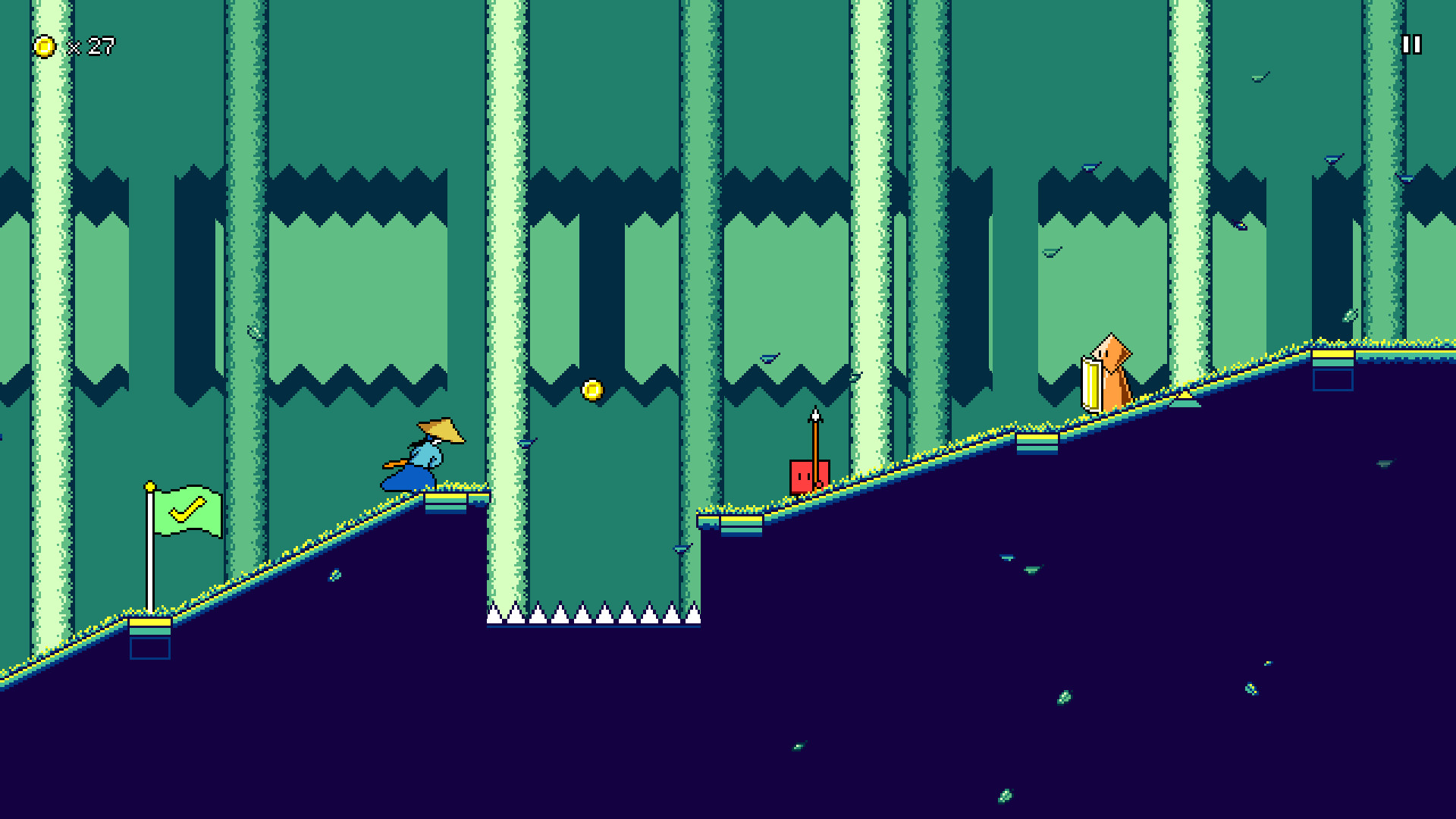
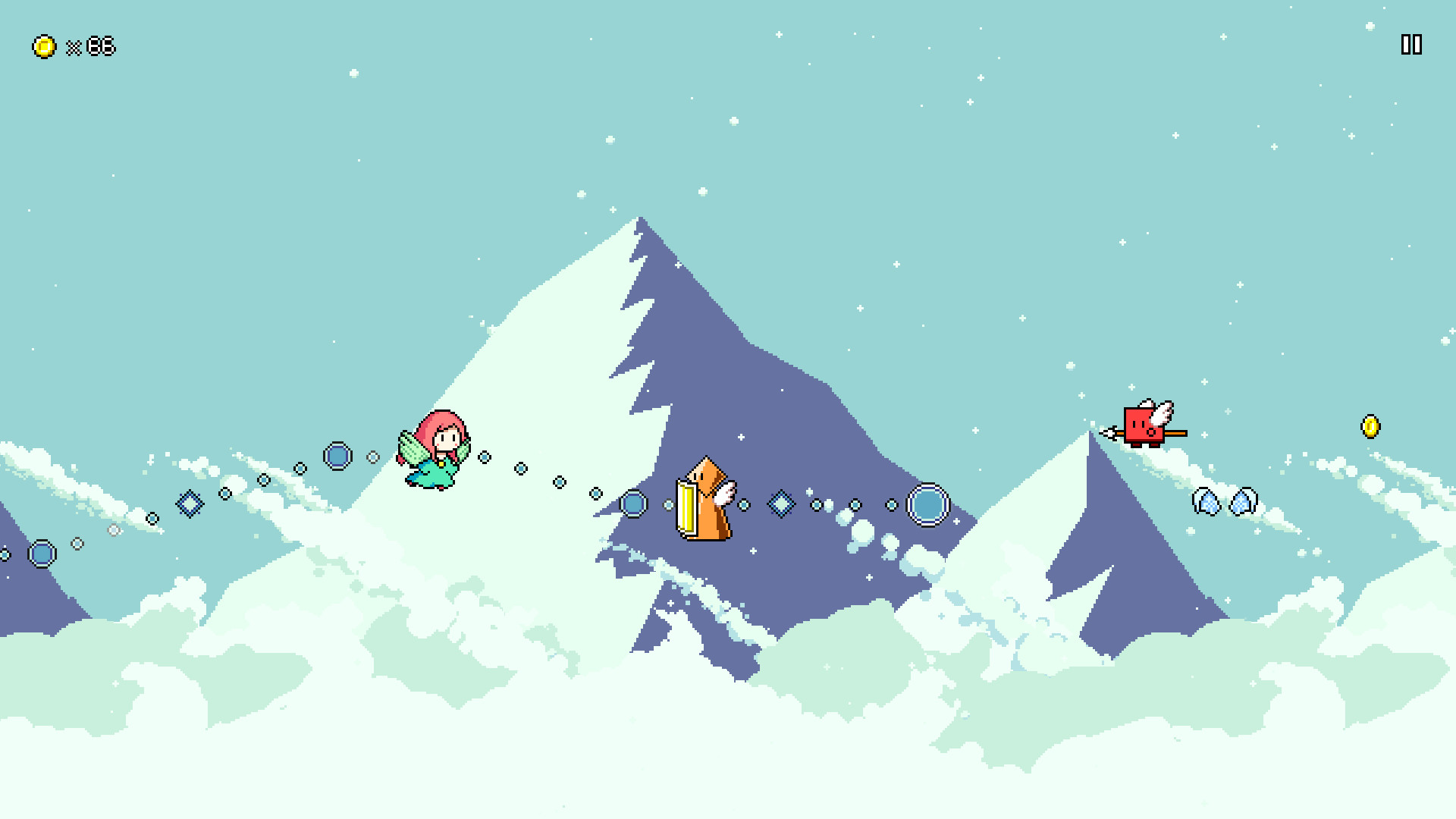
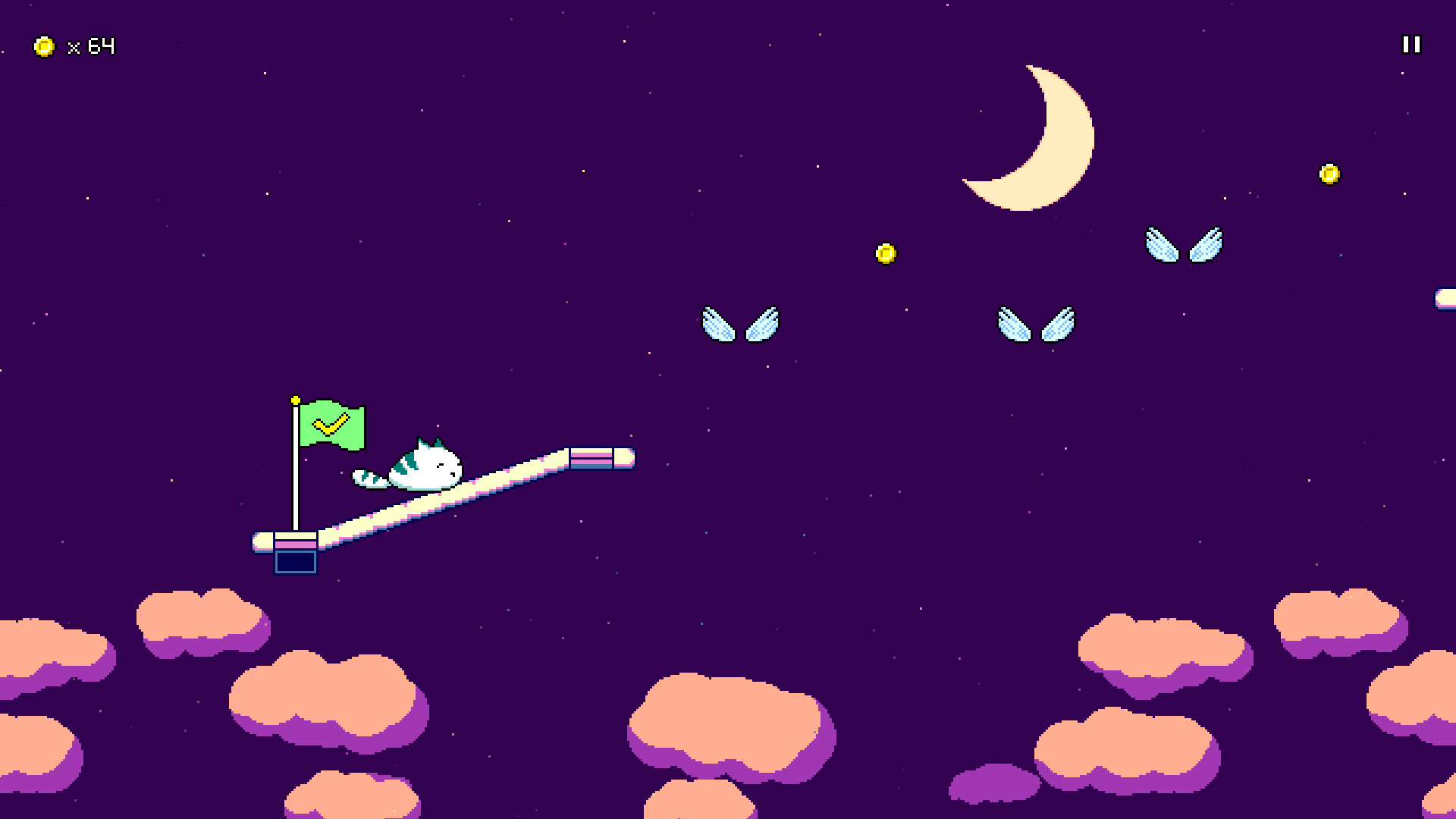
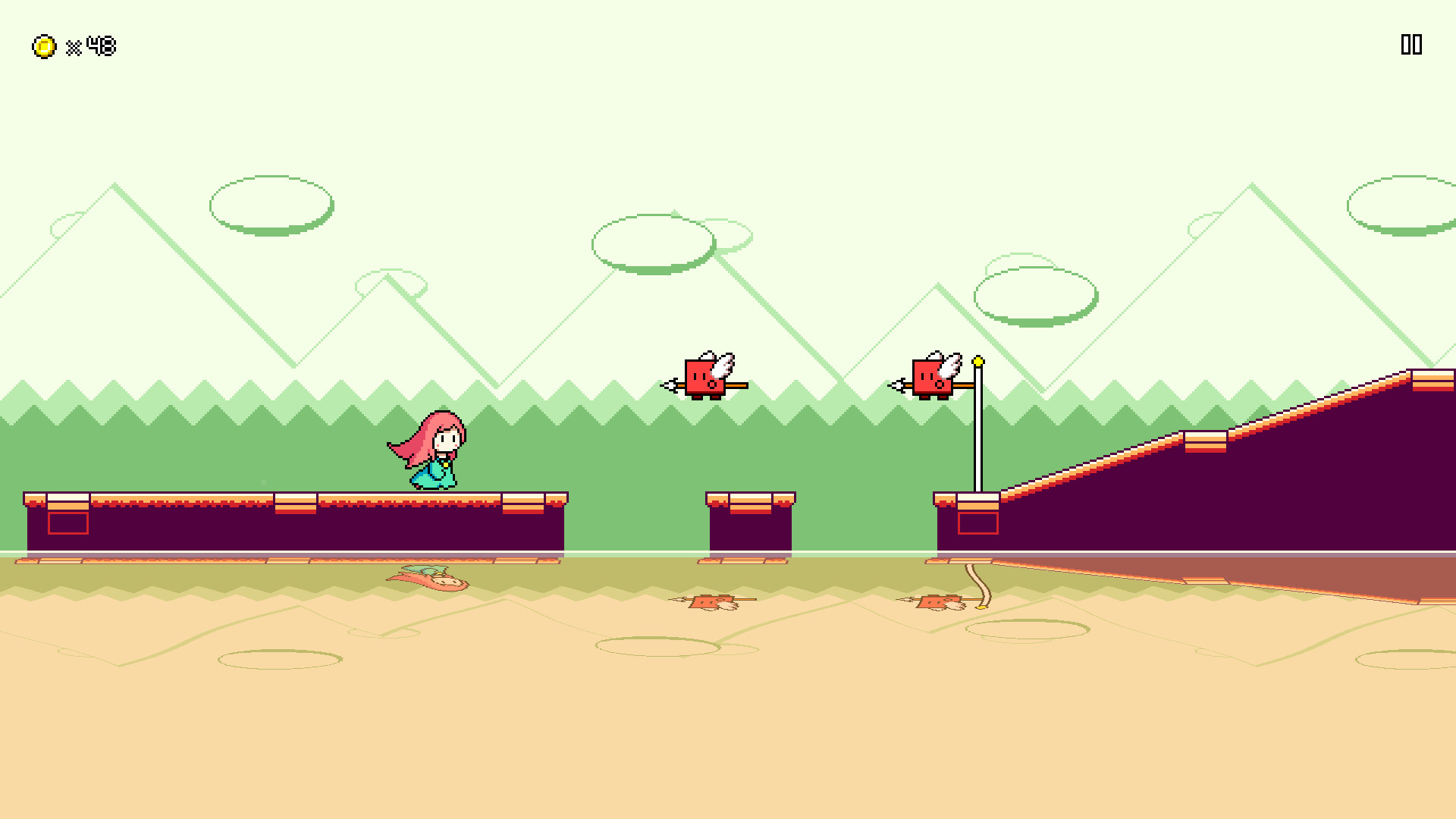
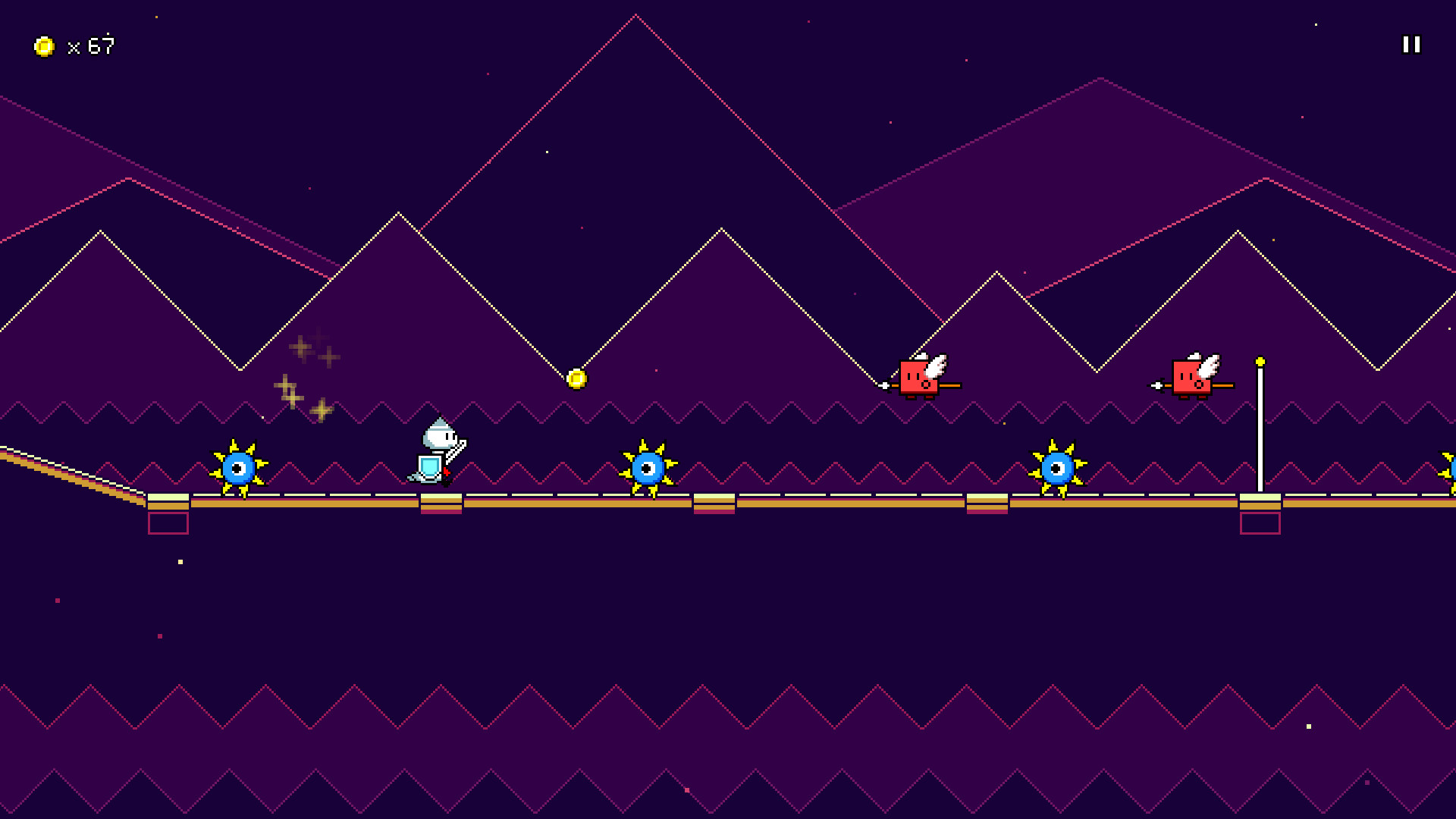
🌟 Special thanks to our amazing supporters:
✨ $10 Tier: [Geeks Love Detail]
🌈 $5 Tier: [Benedikt][David Martínez Martí]
Everyone's favorite thing to see (?) -- new levels! I had a bunch of different life things to tend to this past month (and the rest of Spring is going to be no different...) but I managed to crank out some more charts while in the midst of travelling. Here's a video of those in action:
[previewyoutube=B48lm7YV4cg;full][/previewyoutube]
Scherzo of Magical Science is a whimsical song initially released as part of the Hero Hours Contract OST. At 155 BPM, it's quick, but not blazing fast.
There's a really distinctive 16th note pattern (using a whole-tone scale) at the beginning of the main musical riff. It's too fast for my tastes to chart each of those notes (at least for an official chart), so I ended up using the green attack+jump combo enemies along with flight paths to accentuate that rhythm instead.
Claustrophobia is a song off of the Nyamo's Adventure OST. It's written at 90 BPM, but charted at 180, making it the fastest chart I've made so far.
Again, there are actually some 16th note patterns in the music that you could theoretically add here, but I'm pretty committed to making sure that extremely quick double-taps are not required for any of my charts. Those become more doable when using multiple keybinds/buttons for attacking, but that's just not something I want to get into.
The Warrior Wakes in the Sunrise Dawn is a guzheng piece that I made in an hour, featured in the All in a Day's Work 5 album. This one had to be edited to get rid of the freeform solo portion in the middle, but the rhythms in the rest of the song still feel nice to play through.
The hard version of the chart features some speed zone runs that are difficult but satisfying to pull off. I've had some mixed feelings about the speed zones from time to time because their design space is a little limited, but using them sparingly for fills like this seems to work really well.
Along with the new charts, I spent some time finally pushing out a new release of the demo, which includes most of the new functionality I've been working on in the past months, such as the level browser menu, the new tutorial, and the big scene/level loading refactor. Unfortunately, between the new tutorial and the level load rework there were a big batch of bugs that I didn't catch. Turns out testing the behavior of re-used object instances requires you to actually play through more than just one level at a time, whoops!
I've also added the option to display the diamond-shaped offbeat markers (or downbeat markers, if you configure them that way) on enemies as well as winged jumps, in case that ends up being helpful. I don't think I'm a big fan of having these on everything as a default, so the initial setting is just to have it on wings that are on offbeats. As I said in a previous devlog, these are honestly primarily intended to help with level 2-1, so if they don't end up doing that job, I might end up having to come up with a different solution.
There's still some bugfixes to be ironed out here and there, but besides that I don't have any big aspirations for the next month given my busy schedule outside of Rhythm Quest. Just working on =something= is going to be enough for me to call it a success, I think, but I guess there are one or two specifics that I might try to complete...
The Rhythm Quest Demo has been updated to version 0.36.4. This bugfix patch (along with the previous few) addresses various lingering issues present in the initial 0.36.x release.
Full changelog:
Version 0.36.4
- Fixed checkpoint scheduled sounds not playing on subsequent level loads
- Fixed inconsistent input timeline on subsequent level loads
- Fixed inconsistent obstacle coloration on subsequent level loads
- Fixed inconsistent coin count for level 1-1
- Fixed wrong keybinds showing for tutorial when using custom keybinds
- Fixed UI binds (e.g. arrow keys) not working for custom keybinds
- Hide tutorial icons when skipping level 1-1 tutorial
- Added up arrow as a default keybind for Jump
- Fix pixel artifacts for level tilesets via uv clamping
- Fixed bug when pausing game immediately on level load
The Rhythm Quest Demo has been updated to version 0.36.0! This patch includes a number of changes and improvements all around, including a reworked tutorial sequence, offbeat markers for wings, practice mode improvements, and the addition of the level browser menu.
Full changelog:
Version 0.36.3
- Fix additional level load issues
Version 0.36.2
- Fixed WebGL level load issues
- Fixed WebGL input timing issue
Version 0.36.1
- Fixed various bugs with the 0.36.0 release
- Fixed level 1-1 tutorial for easy/hard difficulties
- Fixed autoplay interactions with level 1-1 tutorial
- Fixed input not being blocked during world 1 banner
- Fixed ui layering issues
- Fixed various level load issues when reusing obstacle instances
Version 0.36.0
- Added level browser menu with streaming previews of all songs
- Reworked level 1-1 tutorial sequence
- Add toggleable diamond markers for obstacles that are not on downbeats
(enabled by default for wings only)
- Tweaked hard chart for level 1-1
- Increased scroll speed for level 2-1
- Add shortcuts for warping to checkpoints in practice mode
- Allow increasing speed beyond 100% in practice/assist mode
- Hide input timeline in practice mode if helpers are disabled
- Fixed coins not reappearing after exiting practice mode
- Spawn (fake) enemy coins in practice mode
- Fixed ugly pixel artifacts in backdrop rendering
- Fixed audio not playing when exiting practice mode
- Slightly reduce sfx/ui volumes in comparison to music volume
- Fixed bugs involving pressing multiple jump inputs for a flight path
- Use pre-existing pitched coin sfx for different music speeds instead of modifying them
- Better updating of scheduled sounds when game settings are changed mid-game
- Update pitch of metronome when music speed is changed
- Major refactor of scene loading implementation
- Reuse obstacle instance between levels to reduce load times
- Major refactor of particle system implementation
- Resize unicode pixel font, force smoothed version at low resolutions
- Updated localizations/translations
- Decreased audio quality for WebGL builds to decrease download size
Last week I completely broke the tutorial setup for level 1-1 and while I was thinking about the best way to fix it up, I figured that I may as well just rework the tutorial since I've been wanting to revamp it for a while anyways...
I planned on making an update to the Rhythm Quest demo to release the new level browser menu, but I got caught up in more work and ended up embarking on a different big refactor that'll be rolling into the same update. It'll be a big one! Nothing too visibly exciting, but cleans things up a lot implementation-wise, and enables some new functionality as an added bonus (which y'all will probably be more excited about than the actual refactor)...
Welcome to 2025! Hopefully this year brings a bunch of more exciting progress on Rhythm Quest...we're going to kick things off with a new bonus level -- this one is called "Bottled Up"! This is actually a song I made all the way back in 2019 for an as-of-yet-unreleased album...unfortunately some of my other music projects have had to take a backseat to Rhythm Quest development, but hey, it's cool that I unexpectedly get to bring this song out in a different way. Here's the playthrough video: [previewyoutube=uVib3To51cU;full][/previewyoutube]
The Rhythm Quest Demo has been updated to version 0.35.0! This patch includes two new difficulty levels for you to try out -- play on Easy mode for a more relaxing experience, or try out Hard mode to challenge your skills with more difficult charts!
Full changelog:
Version 0.35.0
- Added Easy / Normal / Hard difficulties and corresponding charts
- Added spacebar as a default binding for jump (in-game only)
- Recharted / tweaked some Normal difficulty charts and songs
- Added temporary display of difficulty on level start and end
- Reworked water effect for level 1-3
- Fixed bugs with screen filter shaders
- Change end-of-demo popup to only show automatically on first level 3-4 completion
- Prevent binding the same input to multiple action slots
- Attempt to fix pixel clamping rendering issue
- Fixed minor rendering bug for certain menu backdrops
- Reworked level loading implementation (may lead to faster load times)
- Fixed potential load crash when loading old save data (< 0.29.0)
- Fixed practice mode menu navigation
- Update coin sfx pitch to match current music speed
- Fixed VSync setting button not updating correctly
- Fixed incorrect jump/scroll logic when fast-forwarding through level end
Version 0.35.1
- Fixed respawn bug for certain levels (1-3, 2-1) when using timing window mods
- Fixed pixel font underline offset
We're into the start of the holiday season now! I could either take this opportunity to dive head-first into Rhythm Quest work, or I could instead take it as a chance to rest and recover before the start of the new year...knowing myself, I suspect it's going to be neither of those and I'm just going to continue onward at a slow and steady pace!
No fancy new level to show you all this time, but I've been doing good work regardless this month. I have a silly little -- ok, maybe it's really not little anymore, actually quite the opposite -- Trello board where I track tasks and work items that I accomplish for the game, divided into weeks, so you sorta track the number of things that I end up tackling per week. This past month had some columns that are longer than can fit in my screen...
The Rhythm Quest Demo has been updated to version 0.34.0! This patch includes some major internal improvements, including a major Unity update, better gamepad support, performance optimizations, and more!
Full changelog:
Version 0.34.0
- Updated to Unity 6 (removed Unity splash screen...)
- Reworked rendering for menu backdrops to improve performance, especially during transitions
- Other performance improvements
- Add alternative gamepad support to improve gamepad compatibility
- Decreased coin sfx volume
- Loading indicator now matches selected character
- Attempt to handle audio device changes/resets in menu scene
- Fix softlock on changing audio device/improve audio reset behavior
- Fixed spike enemies not responding to skin changes immediately
- Fixed transitions for non-tiling backdrops in low-quality graphics mode
- Localization/text updates and fixes
- Added menu option to skip 1-1 tutorial
- Disabled assist menu during 1-1 tutorial
- Fixed track freezing causing softlock in 1-1 tutorial
- Reset menu backdrop scrolling on transition
- Potential rendering fixes
- Fixed unintended menu description label changes during transitions
- Fix rendering on unicode pixel font by making it much larger
Level 6-4 is sickkk. [previewyoutube=kpZHFdCXuX8;full][/previewyoutube]
Working on new levels has consistently been my favorite part of Rhythm Quest development! As I continue to flesh out my roster of bonus stages (I've got 15-20 at this point!), I've been thinking about other musical styles that I haven't yet represented. One of those is DnB (Drum and Bass), so I made a track called "Midnight Moon" inspired by "Liquid DnB" soundscapes:
[previewyoutube=Xr3riHuEvTU;full][/previewyoutube]
This one is at a very brisk tempo of 170 BPM, but doesn't feature any of the mechanics from worlds 4-6 (water/speed zones, green enemies, yellow ghosts), so it's a good exercise in simply testing your ability to read basic patterns. I'm making more liberal use of syncopated rhythms in the bonus songs throughout, though, so they are going to be a little trickier to sightread than the main stages.
As I've probably mentioned in the past, even when I do use offbeat rhythms, I'm trying to keep them relatively readable, often favoring airjump patterns over basic enemies as they tend to be easier to read at a glance based on height differences.
For the music side of things, the basic structure of the DnB soundscape is actually not very complicated. The foundation consists, of course, of a nice drum loop, and then a prominent low bass synth with a little bit of "wetness" (growl/detune/warble) to it:
https://rhythmquestgame.com/devlog/70-drumsbass.mp3
The cool thing about the liquid DnB-inspired soundscape for me is that the tight drums contrast with the other melodic layers going on, which are either drenched in lush reverb, or have softer textures. Here I'm using a droning bell-type pad loop sample, some electric piano-ish plucks, and then a chiptune arpeggio that's put through a ton of reverb:
https://rhythmquestgame.com/devlog/70-texturelayers.mp3
Here's all of that coming together in the main drop of the song, which also adds in a few other new elements (an additional drum loop layer, a high blippy synth):
https://rhythmquestgame.com/devlog/70-drop.mp3
The Rhythm Quest Demo has been updated to version 0.32.1! This patch contains a few bugfixes, but also finally allows you to pause during the middle of a respawn.
Full changelog:
Version 0.32.1
- Allow pausing during respawn (finally)
- Fixed pause music continuing to play after exiting practice mode
- Fixed music desync when pausing during level 1-1 tutorial
- Attempt to handle audio device resets/changes by restarting music
- Fixed minor menu navigation issues
- Fixed some text strings
The Rhythm Quest Demo has been updated to version 0.32.0! This patch adds in various assist features to help if you're struggling with particular levels or sections of the game.
Full changelog:
Version 0.32.0
- Added track preview, practice mode, track freeze assist features
- Added assist prompt when failing a section too many times
- Added control settings to in-game menu
- Added setting to enable/disable coin collection chime
- Tweaked/increased respawn timing
- Increase coin loss on respawn
- Decrease coin bank amount on checkpoint
- Fixed pause drum loop played after unpause
- Fixed pause drum loop synchronization issues when music speed was altered
- Fixed missing blur screen filter description
- Fixed jump arc calculations
- Fixed doublehitenemies always awarding coins even after respawn
- Fixed wrong ghost helper beat-matched sprite colors for second helper
- Fixed beat-matched colors not updating correctly via in-game menu
- Fixed visual hitch for ghost helpers on respawn
- Fixed keyboard rebinding not being available on web build
- Updated localizations
Another month, another new level. Hopefully I can at LEAST keep this pace up, if nothing else, haha. There's some other stuff I worked on that's not ready to show off yet, but here's the preview for level 6-3, titled "Quantum Orbit": [previewyoutube=irJiF6vO960;full][/previewyoutube]
New levels! In addition to charting out "Song of the Sea (Expert Mix)" from Melody Muncher, I've added two Mozart compositions to Rhythm Quest. Here's the video showing off some excerpts from those levels: [previewyoutube=ZGWfzXa-7y4;full][/previewyoutube]
The Rhythm Quest Demo has been updated to version 0.31.0! This patch includes bugfixes and some new mod options, including the ability to switch the color mapping for obstacles in levels.
Full changelog:
Version 0.31.0
- Added color skinning visual mod settings to aid readability
- Added visual mod to adjust horizontal placement of enemies
- Added timing mod to scale timing windows by music speed setting
- Added visual mod to re-show collected coins after respawn
- Tweaked respawn timings, especially across different music speeds
- Fixed not being able to buffer jumps out of pause
- Fixed ghost enemies not respawning quickly enough when offscreen
- Fixed some level select logic when levels are played out of order
- Fixed flight paths jumps pulsing with incorrect beat offset
- Minor backdrop scrolling tweaks
- Fixed mis-sized character shop graphics
- Fixed checkpoint activation sounds sometimes replaying on respawn
- Fixed missing coin animation frame
Well, first things first -- I've got a new level to show off! I decided to move the speed zone level (formerly 6-1) to become level 6-2, and instead have this new level 6-1 which introduces the yellow ghost enemies in their basic state. Here's the video: [previewyoutube=p8gRCw3QZMc;full][/previewyoutube]
Incredible - Rhythm Quest now has over 20,000 wishlists on Steam!
I just wanted to take a moment to thank you so much for all of your support and interest...I'll continue doing my best as I work towards a full release!
Another month, another devlog. At least I'm consistent, if nothing else. That consistency is what I've relied on for the past 8 years of development (has it really been that long!?), so hopefully it'll continue to carry me through.
It's been a while, so I definitely have some cool stuff to show off from this past month of work! Let's start with everyone's favorite, looking at some more new levels...
Sometimes it can be good to bounce around and work on some different things here and there. This time I worked on some additional levels! I'm still feeling hesitant to finish out the levels in world 6, so I decided to work on some bonus levels instead. This is relatively low-pressure for me since the more bonus levels I have, the better, and they don't really have to fit a particular level of difficulty or anything. I also wanted to explore using the new yellow ghost enemies a bit to get to know how to chart with them.
I decided to explore the mod.io integration that I mentioned last month, and have decided to move forward with it, but I was getting a little overwhelmed trying to figure out exactly how I wanted to hook up all of the export/import/login flows, so I decided to table that and work on more core level editor functionality instead.
The Rhythm Quest Demo has been updated to version 0.30.0! This patch includes some small but important improvements as well as a number of bugfixes for community-reported issues. The game internals also received a refresh with a new Unity version as well as some plugin updates (hopefully not breaking anything!).
I forgot to list it in the official changelog but this build also fixes a critical issue on later versions of Mac Sonoma where the game was unresponsive to inputs.
Full changelog:
Version 0.30.2
- Fixed scheduled sounds not playing correctly after respawns
Version 0.30.1
- Fixed wrong version of some east asian glyphs being used
- Fixed broken "Audio Mods" menu in main menu scene
Version 0.30.0
- Updated Unity version
- Fixed issue on later Mac Sonoma versions where the game was unresponsive
- Added pitched coin/metronome sfx to match the key of each level
- Added ability to fast-forward endlevel sequence by holding menu button (escape)
- Fixed "bumpy" camera movement across ramps/beatgrids
- Fixed missing default keybinds for 'B' and '/'
- Re-fixed certain accessibility audio cues sometimes not triggering
- Fixed blurry horizontal line when using smooth font in shop display
- Tweak line height for unicode pixel font
- Tweak font pixel snapping logic
- Camera pixel rendering fix for odd-pixel resolutions
- Fixed offbeat pulse timing on respawn
- Replaced and refactored Steam integration
- Running the Steam build outside of Steam will no longer restart the game via Steam
- Allow changing resolution in exclusive fullscreen mode
- Added several more windowed resolution options for large displays
- Fixed incorrect description for "Blur" screen filter
- Fixed demo end screen showing up incorrectly after data reset
- Fixed menu pulse animation speeds sometimes being incorrect
My most recent work on custom levels has been this screen where you can import new custom levels, either ones that you've already downloaded, or by typing/pasting public URLs where they're hosted:
Internally this importer needs to handle a bunch of different things -- fetching the level package, making sure that it doesn't already exist in your levels folder, and extracting the appropriate files from the archive. It's all more or less working now, though the "Browse Steam Workshop" button just takes you to an empty page (more on that later)... It currently also supports file drag-and-drop, though only on Windows (sorry, this is a really OS-specific thing and I couldn't find any other implementations!).
It'll probably be good if I can try to write my devlogs more concisely so they don't feel like such a chore to post...let's just get right into it.
The Rhythm Quest Demo has been updated to version 0.29.4! This patch features more bugfixes and adds a quality-of-life improvement for input bindings that should help players who are using high speed mods. Full changelog: Version 0.29.4 - Multiple key/gamepad bindings for the same action now function independently to allow for easier multipresses - Added "blur" background filter - Fixed keyboard button focus being lost after closing menu overlays - Fixed jump logic when overlapping multiple obstacles - Fixed jump miss sound playing on hit when sound scheduling disabled - Fixed certain accessibility audio cues sometimes not triggering - Fixed doublehit enemy inputs sometimes not respawning on input timeline - Added support for localizing "Congratulations" text on demo end - Slightly tweaked initial camera pan on load
Oops, I missed my monthly devlog post for November. On the plus side, I've been doing a lot of good work over the past few weeks! I've found that my time and motivation for Rhythm Quest tends to oscillate a little bit over time -- sometimes it's because other parts of my life are busy, other times it's because I'm working on something that's tougher to get motivated about. But I do my best to just keep plugging away slowly and steadily... It's a little crazy to think that ~3 months ago the Rhythm Quest level editor didn't exist at all! Now we've got an entire editor tool palette UI, notifications, undo/redo, saving and loading, input timelines, waveform displays, animated preview sprites, automatic beat detection, level validation, ... There are like, infinity different things that go into custom level support, which is why I initially wanted to put it off until post-release...but I'm actually having a lot of fun developing it all, so that's great!
Level | Actions/Second | Linear Difficulty | Arctan Difficulty
1-1 | 0.859375 | 17 (Easy) | 3 (Easy)
1-5 | 1.560284 | 31 (Medium) | 9 (Easy)
2-3 | 1.718750 | 34 (Medium) | 11 (Easy)
2-5 | 1.984972 | 40 (Hard) | 17 (Easy)
3-4 | 2.195684 | 44 (Hard) | 20 (Medium)
4-5 | 2.761905 | 55 (Hard) | 41 (Hard)
5-5 | 3.345877 | 67 (Expert) | 70 (Expert)
| 4.000000 | 80 (Impossible) | 93 (Impossible)
| 5.000000 | 100 (Impossible) | 100 (Impossible)
Of course, this is just a first attempt, so it's definitely possible that it'll need some tweaking or shifting around...
It feels like I've been really inconsistent with these devlog entries, but looking back it seems like I have been basically putting out one per month quite regularly, so maybe it's not as haphazard as I thought!
The Rhythm Quest Demo has been updated to version 0.29.2! This patch features some more bugfixes, but also adds a few in-game mods including an input timeline and metronome. Full changelog: Version 0.29.3 - Tweak/fix grass in world 1 level select Version 0.29.2 - Fixed a softlock bug that allowed getting stuck in walls - Slightly lowered audio encoding quality to reduce build size - Fixed incorrectly-centered vertical backdrop scaling behavior - (This fixes an issue where the foreground in 3-1 could cover more than expected) - Fixed a bug where keyboard menu selection was not restored for certain menus - Fixed a bug where the player could run off the level if the game is paused after a level end - Fixed perfect medal animation offsets in world select for demo version - Added "Run in Background" setting - Added "Input Timeline" visual mod - Added "Metronome" audio mod - Fixed tutorial being skipped if 1-1 is restarted during it - Fixed scheduled sounds being very slightly offset in some cases - Fixed end level medal particles having different speeds depending on resolution - Allow pressing any key in calibration screen (not just space) - Fix subpixel render misalignment of player in game - Fix subpixel render misalignment of ground in menu - Disabled screen refresh rate label (currently not working) - Localization updates
I've been continuing to try my best to clock in some hours toward fleshing out the Rhythm Quest level editor! Here's some quick demos of what is now possible in the editor:
I've been kinda radio-silent over the past month. The first part of that was due to simply not getting that much done, but in the latter half of the month I had a better (and more exciting) reason for foregoing updates: I've been hard at work building out the initial skeleton of the Rhythm Quest Level Editor!
"Obstacles": [
"#... .... *... *... *... *...",
"#^.^ .-11 .^.^ .+11",
"#+.- .*.* .+.- .*.*",
"#.11 ..^1 ..^^ 1111",
"#*.* .*.* .111 1.11",
"#.^^ ..** ..^^ ..**",
"#.^^ ..** ..^^ ..**",
"#1.+ .1.^ .1.- .11*",
"#1.+ .1.^ .1.- .1**"
],
The different symbols here are a representation of different types of events. '#' represents a checkpoint, for example, while '1' is a basic enemy, and '^' is a normal jump. ('*' is shorthand for a jump followed by a flying enemy.) When the engine parses this string, it converts it into its respective sequence of timed events, so something like:
_events = {
new EventData(0.0f, EventType.Checkpoint),
new EventData(8.0f, EventType.NormalJump),
new EventData(8.5f, EventType.SingleEnemy),
new EventData(12.0f, EventType.NormalJump),
new EventData(12.5f, EventType.SingleEnemy),
...
};
This (along with other metadata about the level) then gets passed off to the level generation procedure, which is responsible for processing all of the events in order and building the actual level out of them. Normally this is all done ahead-of-time when I author the levels (in a process I call "baking" the levels), so the end level objects are saved into the scenes directly to optimize load time.
Now, the way that the (work in progress) level editor works is simply by maintaining a similar list of events that compose the level being edited, and re-generating the level again every time there's any change. It might seem terribly inefficient to keep rebuilding the level compared to just editing the resulting level objects directly, but there's a lot of reasons why it makes sense to do things this way. For example, changing a list of events is simply more efficient than having to worry about editing the actual level objects (moving floors around, etc). and I already have the code to do all of this, so I just have to worry about providing an interface to visualize these changes well.
The Rhythm Quest Demo has been updated to version 0.29.0! This patch fixes a bug with the latency calibration measurements (you may want to re-calibrate) and reworks the coin/medal system, along with other fixes and improvements. Full changelog: Version 0.29.1 - Fixed localizations not falling back to English translation - Modify respawn/fadein timing for enemies according to music speed/respawn timing - Fixed steam build not running properly when started outside of steam - Localization updates Version 0.29.0 - Fixed auto-calibration output values (was previously ~75% too low) - Reverted level medals to be based on coin count again - Changed coin thresholds for medals (25%, 50%, 75%) - Changed coin loss amount/mechanic to be much less punishing: 50% of non-banked coins are now "banked" on each checkpoint and can no longer be lost 50% of non-banked coins are lost with each respawn - Added new level end coin/medal display - Updated ghost teleport to change speed based on music speed mod - Updated input handling interactions to be more timing-accurate - Fixed an input bug triggered by having multiple inputs on the same frame - Fixed a bug causing the color invert fx on doublehit enemies to persist - Fixed a timeslicing issue that sometimes caused interactables to be skipped at high speeds - Try (again) to fix rendering issues at odd resolutions - Added Respawn Timing setting - Fixed ghost enemy trail effect to render consistently regardless of framerate/speed - Fixed screen refresh rate label not actually updating - Localization and UI updates - Fixed respawn count not resetting properly when checkpoints are disabled - Fixed the ability to finish a level and respawn simultaneously
The Rhythm Quest Demo has been updated to version 0.28.0! This patch includes a multitude of extra settings and game mods, including the ability to play levels at a faster or slower speed! Full changelog: Version 0.28.0 - Fixed ramped floors being allowed with spike enemies - Renamed "Cheats" menu into "Game Mods" - Added assists/game mod settings to in-game menu - Made shop accessible from Extras menu - Added music speed game mod setting - Added timing window game mod setting - Added ghost helper game mod setting - Added frame queueing graphics setting - Added configurable bindings for gamepad controls - Keyboard bindings now default to entire left/right half of main keyboard area - Fixed rendering artifacts at odd resolutions - Added UI volume setting, separate from sfx volume, reduced UI sfx volume slightly - Change pixel font setting default based on language at runtime - Added low quality graphics toggle to help framerate for older devices - Added "Released Early" and "Released Late" text for hold presses - Added "screenreader" command line argument force start with screenreader prompt - Fixed too-fast scrolling before level 1-1 tutorial - Fixed fullscreen not working for devices that present resolutions in an unexpected order - Fixed vsync off behavior, especially for desktop platforms - Changed flashing effects to be time-based rather than frame-based, to support higher refresh rates - Settings are now saved when drilling into a submenu (not only when navigating back) - Progress is now saved (again) while auto play is enabled - Thickened some outlines on smooth text - Minor tweaks to jump logic (hopefully not breaking anything) Version 0.27.0 - Added tracking of respawn counts to save data and level display - Localization and UI updates
Despite what it might seem like, I've actually been working on quite a lot of different things for Rhythm Quest recently! Let's get right into it...
I'm continuing my break from working on world 6 levels for now. I've sent out the current build to some internal beta testers to get some feedback on the difficulty scaling and reception to the newer mechanics, so I want to give myself a chance to let that feedback come in and stew on it for a bit before I continue on with the last 4 levels of the game. In the meantime, I've been trying to tackle some improvements and fixes that have been laying around in my backlog for a while...
The Rhythm Quest Demo has been updated to version 0.26.5! This patch includes some hefty optimizations, which should help improve performance, particularly on lower-end machines. Full changelog: Version 0.26.5 - Reworked texture encoding for memory and performance benefits - Various other performance optimizations - Fixed an issue causing minor vertical blurring on WebGL builds - Add better transition for Furball attack -> jump - Fixed attack animation being cancelled on fly start - Fixed menu bug where foreground level backdrops sometimes failed to fade in - Minor UI fixes
Somewhat unexpectedly, I took a break from working on levels this month to focus instead of **performance and memory optimizations**. This was brought on by the fact that I made some release builds for the first time in a while and found that my iOS build crashed on startup because it was running out of memory loading the main menu!
The main culprit? These huge backdrop texture atlases...(this one is 64 MB!)...
{ "level2-5_background_4", new Entry {
Offset = -62.5f,
TopTransparency = true,
BottomTransparency = false,
OpaqueBelow = 1,
OpaqueAbove = 55
} },
My backdrop tiling script is responsible for taking the stored offset metadata and shifting the center position of the rendered sprite accordingly.
The second issue is that while Unity supports texture coordinate clamping, there's no way to do that when the sprite in question is one of many sprites packed into a texture atlas! Unity's sprite renderer only handles tiling in a very specific way, which no longer applied to what I wanted to do, so I had to modify my fragment shader to handle the texture clamping part.
In order to do this texture clamping correctly, I also needed my fragment shader to understand what UV texture coordinates it was supposed to be working with inside the texture atlas. Normally the fragment shader is completely oblivious of this -- the Sprite renderer is responsible for handing it a set of UVs to render and then the shader just does the texture lookups blindly.
It also turns out that you don't actually have access to the sprite UV metadata from within your fragment shader =/. So I needed to pass those into the shader, =and= I couldn't use uniform variables since that would break batching. Luckily, Unity happens to expose a SpriteDataAccessExtensions class which allows you to write to the UV texture coordinates of the sprite mesh used by a sprite renderer internally.
In addition to allowing you to modify the main UVs, it also lets you set additional texture coordinates on the mesh (TexCoord1, TexCoord2, TexCoord3, etc.). I used those to pass extra data to the vertex shader -- and then through to the fragment shader -- including the sprite UVs from the texture atlas.
This took a lot more debugging to get right, but at the end of all that, it was working! Here's the new version of the texture atlas from before (in all its red-channel glory), which is 1024x1024 instead of 4096x4096, and 1 MB instead of 64 MB!
I'm continuing to just roll ahead with levels! It's funny, I feel like there was a long period of time when working on new levels and thinking about the mechanics felt intimidating, so I would just procrastinate on it and work on other miscellaneous things. But now I think it's the opposite (probably partly because all of my mechanics are known now), where I've gotten into the habit of just working on only levels. It's good though, the levels are something that need to be done 100%. Anyways, I went straight ahead and finished up the first level in world 6, level 6-1! [previewyoutube=BWk1T_4gv1w;full][/previewyoutube]
Despite not having a lot of time/energy to throw around this month, I somehow managed to finish working on level 5-5, tentatively titled "Brilliant Boulevard":
[previewyoutube=PvyRg6PRPMw;full][/previewyoutube]
As is usual with the last level in each world, there are no new mechanics here, so it's just combining everything from the previous levels and amping up the difficulty. The tempo is significantly faster than the slower-paced level 5-4, which makes the chart a lot more dense in terms of inputs.
For visuals, I experimented somewhat aimlessly with different shapes until I settled on this sort of "spiked" design, mirroring the top and bottom so it kind of feels like stalactites and stalagmites. It's reminiscent of the design from level 5-1, just with different shapes. Again my lack of visual "complexity" is showing here with the simplistic shapes -- the visual detail really lies in the layering (translucency!) and parallax scrolling.
I also experimented with having syncopated / offbeat spike enemies in the tail end of this level (so far they've only been on downbeats). Normally this is a little hard to read, but adding the green enemies actually makes it fine since it gives you a static marker to read the rhythm (even if the spike enemies were invisible you could still play this section fine):
This is one of those curious instances where adding additional obstacles (having green enemies, instead of just rolling spike enemies) actually makes the chart easier, not harder. There are many types of possible difficulty in Rhythm Quest charting ("random" notes that lack patterns would be incredibly awkward and difficult to read), but I'm following rather specific charting philosophies in order to feature difficulty in the "right" ways -- at least, for the main campaign.
That wraps up the entirety of world 5, which means next up I'll have to find some sort of musical and visual identity for world 6, where I'm supposed to introduce triplet-based speed zones!
The bad news is that it's been 2 months since my last devlog post! The good news is that I've finished 3 entire levels in that time: [previewyoutube=Yy4lgDCAz-o;full][/previewyoutube] [previewyoutube=D4HXrDVYopE;full][/previewyoutube] [previewyoutube=IGpfwxg46F8;full][/previewyoutube]
The Rhythm Quest Demo has been updated to version 0.26.3! This patch fixes accessibility cue volume and makes some other minor tweaks. Full changelog: Version 0.26.3 - Fixed audio cue volume balance not affecting music/sfx volume - Slightly increased saturation for wing sprites - Fixed enemies sometimes fading in suddenly on respawn - Fixed minor texture rendering issue during respawns - Fixed level 1-1 tutorial not respecting screen filter - Changed menu player control behavior - Minor menu tweaks
I've completed work on level 5-1, tentatively titled "Gleaming Glassway"! This is the first level in world 5 (Crystal Caverns). I've got a =ton= to talk about for this level, but first, here's a video of a full playthrough of it: [previewyoutube=bodJ8_mIvuc;full][/previewyoutube]
The Rhythm Quest Demo has been updated to version 0.26.1! This patch implements some minor bugfixes and quality of life tweaks, as well as updated Sayuri sprites and a new Vietnamese localization. Full changelog: Version 0.26.1 - Updated Sayuri sprites to properly feature her ears - Added "Perfect Clear Mode" / disable checkpoint setting in cheats - Auto play mode no longer saves progress - Buffer pause inputs during respawn - Added Vietnamese translation - Fixed bug that prevented click input on initial calibration screen - Select TTS voice based on language for OSX - Allow/implement pausing during level 1-1 tutorial sequence - Fixed position warping bug when game loses focus or fails to update
The Rhythm Quest Demo has been updated to version 0.26.0! As thanks for reaching 10,000+ wishlists on Steam, this update includes a brand new level in the demo, level 3-4, which features the purple "ghost" enemy mechanic!
Full changelog:
Version 0.26.0
- Made level 3-4 avilable in the demo!
- Added missing pixel font diacritics for Polish
- Fixed Chinese, Ukranian localizations not forcing smooth font for low resolutions
- Save data validation tweaks
- Text wrap/padding tweaks
- Fixed doubled web browser link opens
- Fixed calibration squares sometimes not flashing
- Tweak graphics settings submenu layout
- Prevent mixing of different pixel fonts for unicode/non-unicode glyphs
- Keyboard bind displays now account for keyboard layout
Happy holidays, everyone! I've got another new level to show off today, but before we get into that, I'd like to celebrate the awesome milestone of hitting 10,000 wishlists on Steam!
I'm really astounded by how much traction the game and demo have gotten so far (despite being admittedly mediocre at effective promotion). Of course, Steam is not the only platform that matters to me (iOS, Android, Switch, itch.io), but it's the one with the most defined marketing metrics, so it's a nice way of getting a pulse on interest.
As a celebration and thank you for hitting 10,000 wishlists, I'm planning to add one more level in the Rhythm Quest Demo soon -- probably something like level 3-4 that involves the multi-hit ghost enemies. That would bring the demo content to 9 levels in total. There are 30 levels in the game, so that is actually quite a fair bit, but I don't think I mind too much. I will have to figure out how to make the demo work with including levels 3-1 and 3-4 but not the levels in-between (ick...) so that might take a little bit, but I'll try to work on it at some point.
The Rhythm Quest Demo has been updated to version 0.25.1! This release includes several new languages, including Italian, Polish, Simplified Chinese, Swedish, and Ukranian -- big shout out to the community translators who made these localizations possible! Full changelog: Version 0.25.1 - Fixed mouse input not working in calibration screen - Text padding and localization tweaks/fixes Version 0.25.0 - Added several new languages and localization improvements - Accessibility fixes, especially for demo end screen - Expanded credits screen - Attempt to prevent performance hitch when enabling particles - Added diacritics to large pixel font - Fixed respawn count text spacing - Performance improvements
Hello Steam folks!
For those who are unaware, I've been regularly posting devlog articles for a while now, both to Medium as well as the official Rhythm Quest site. Since Rhythm Quest is starting to get more traction on Steam (thank you all!), I'm going to try to start cross-posting those here as well.
Before we start, I've got a quick announcement: I'm now accepting applications for translation heads! If you'd like to volunteer to be in charge of the localizations for a particular language, please go to this form to learn more details and apply!
It's been too long (5 months, somehow...) since I last completed a level, but I'm finally back at it, this time with the second-to-last level in world 4. Here's a video of level 4-4 in its entirety:
[previewyoutube=vlEI6y2bfIs;full][/previewyoutube]
Level 4-4 takes a slightly slower tempo (110 BPM, vs 128 BPM for level 4-3) and cranks it up a notch with the interplay between water zones and air jumps/flight paths. It also specifically leans into a few syncopated rhythms, such as this flight path riff:
One of the "rules" I'm forming for myself as I chart these levels is that syncopation is that off-beat rhythms are mostly off-limits when it comes to attacks or ground jumps because of readability concerns, but they're much easier to interpret when it comes to air jumps and flight paths because the height differentials give you an indication of the relative beat positioning of the notes.
Of course, water zones change up the visual representation of those beat positionings, which is what this level is all about:
Visually, I wanted to do a skyline theme, as I hadn't done that before. World 4 has a little bit of a visual identity crisis at the moment, flipping back and forth between "alien space world" and "cherry blossoms", so I don't know if this truly fits in, but as with a lot of things, I'm just going to roll with it now and question it later.
I was inspired to do this pink/purple color scheme, which feels vaguely scifi-esque to me. There are a bunch of examples I found by other artists of this sort of look (please let me know if you find the original artist for either of these works so I can credit appropriately):
My process was essentially to draw a bunch of layers of semi-random tall rectangles, then afterwards go back and add some sporadic details along the tops of several of them. There isn't actually a ton of detail here -- I decided to just stop after adding a few as it seemed to work well enough. The only other real thing going on is the sun in the very back, and then a bunch of layers of semi-transparent clouds (which are all over the place throughout world 4 -- that part at least is visually distinctive).
The color palette here is pretty straightforward by now: notice how the hue goes from purple to reddish-pink as we fade into the background layers. The colors get brighter and less saturated for the back layers.
Overall I'm happy with how this one turned out (I feel like I say that every time...), but I'm mostly just happy that I still managed to complete another full level. There's only one level left to do for world 4, so I think that'll probably be next on my list...
Hi all! I'll be doing a developer livestream of Rhythm Quest on Tuesday Oct 4, at 6PM PDT/9PM EDT.
You can catch the stream here on Steam, or on Twitch at https://www.twitch.tv/ddrkirbyisq
I'll be going through all of the currently-finished levels, including 10 new stages that aren't included in the public demo!
Rhythm Quest already has a live demo, please check it out on Steam here!
For more information about Rhythm Quest, please visit the website here or watch the gameplay trailer below:
[previewyoutube=vwtPYznqZHg;full][/previewyoutube]
(This event takes place on Tuesday Oct 4. Please disregard the steam event start time) This event is now scheduled for Tuesday Oct 4. Please click here for the updated event page!
[ 6081 ]
[ 1507 ]
[ 1999 ]